Maximizing Efficiency: Unleashing the Power of Async/Await with a Foreach Loop
Discover how to maximize efficiency and performance in JavaScript web development by combining async/await with a foreach loop. Learn the seamless integration of these powerful concepts, optimizing code for scalability and responsiveness. Unlock the potential of asynchronous programming and gain insights into the benefits of this technique. Read our blog post to enhance your coding skills today
DEVFEATUREDQA
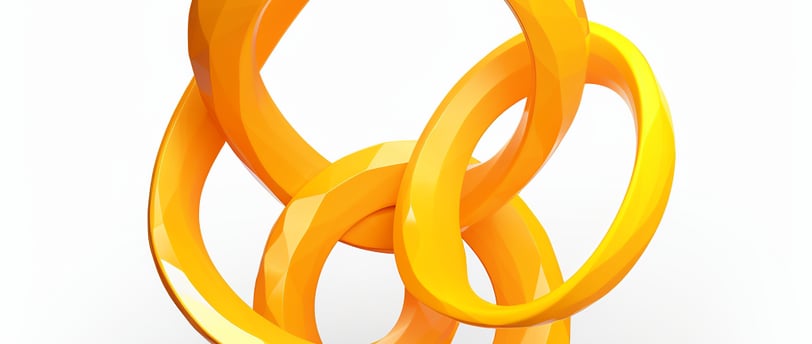
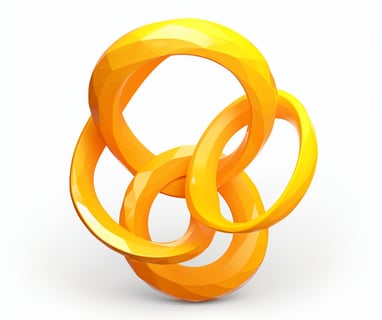
Maximizing Efficiency: Unleashing the Power of Async/Await with a Foreach Loop
In the world of modern web development, performance and efficiency are paramount. Asynchronous programming has become an essential technique to optimize resource utilization and responsiveness. JavaScript's async/await feature is a powerful tool that simplifies working with asynchronous operations, making code more readable and maintainable. This article will explore leveraging async/await with a foreach loop to achieve efficient and scalable code.
Understanding Async/Await:
Before diving into the details of combining async/await with a foreach loop, let's briefly understand the fundamentals. Async/await is a syntax introduced in ECMAScript 2017 (ES8) that simplifies handling asynchronous operations in JavaScript. It allows developers to write asynchronous code that looks and behaves like synchronous code, making it easier to reason.
Working with a Foreach Loop:
The traditional foreach loop is commonly used to iterate over arrays or collections. However, dealing with asynchronous operations within a foreach loop can lead to unexpected behavior. By utilizing async/await, we can overcome these limitations and harness the full potential of both constructs.
1. Using async/await with a Foreach Loop:
To get started, let's consider a scenario where we have an array of items and need to perform an asynchronous operation on each item. We want to ensure that the execution of each asynchronous task doesn't block the loop.
async function processItems(items) {
for (const item of items) {
await performAsyncTask(item);
}
}
async function performAsyncTask(item) {
// Perform your asynchronous operation here
// Example: Make an API call, fetch data, or process data asynchronously
await someAsyncOperation(item);
}
The code snippet above defines two functions: `processItems` and `performAsyncTask`. Using a for-of loop, the `processItems` function iterates over the `items` array. Inside the loop, we await each item's completion of `performAsyncTask`.
2. Handling Errors:
When working with asynchronous code, it is essential to handle errors gracefully. Async/await simplifies error handling by allowing us to use try-catch blocks around asynchronous operations.
async function processItems(items) {
try {
for (const item of items) {
await performAsyncTask(item);
}
} catch (error) {
console.error("An error occurred:", error);
}
}
In the updated code snippet, we wrap the foreach loop inside a try block and catch any errors during the asynchronous operations. This way, we can gracefully handle errors without interrupting the entire loop.
Conclusion:
By combining the power of async/await with a foreach loop, we can achieve efficient and scalable code that harnesses the benefits of asynchronous programming. This approach allows us to handle asynchronous operations seamlessly, improving the performance and responsiveness of our applications.
Remember to optimize your code further by utilizing additional asynchronous techniques and exploring the vast ecosystem of libraries and frameworks available for JavaScript.
In conclusion, embracing async/await with a foreach loop empowers developers to create robust and highly performant applications, all while maintaining clean and readable code. Start leveraging this powerful combination in your projects and unlock the true potential of asynchronous programming in JavaScript.
Note: The code snippets in this article are for illustrative purposes and may require adaptation based on your specific use case.